A simple mobile app for the defunct dartboard Darts Connect
Built with Kotlin Multiplatform (KMP) and Compose Multiplatform (CMP) for Android and iOS
A while ago a friend gave me his old electronic dartboard. The model is “Darts Connect,” a 2016 Kickstarter and Indiegogo project. The board connects to an Android app via WiFi, allowing you to play locally or over the internet with other users. I believe an iOS version was also planned, but never released. Unfortunately, the company doesn’t exists anymore and the app doesn’t work on newer Android versions.
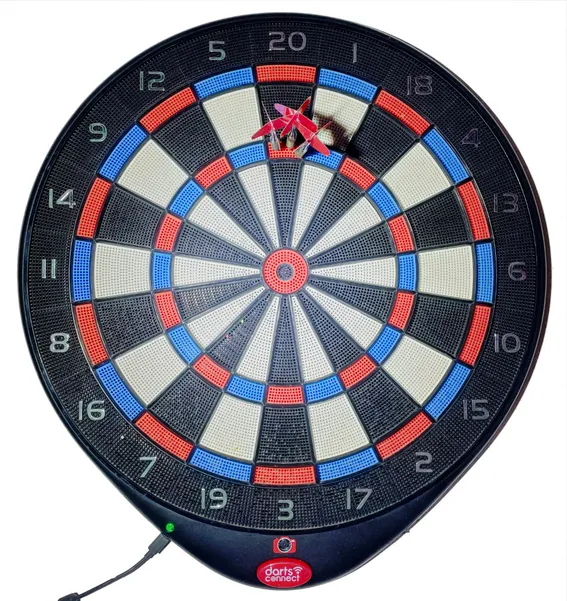
So I decided to make my own app. I also wanted to experiment with Kotlin Multiplatform (KMP) and Compose Multiplatform (CMP), and this project seems like a perfect fit. The source code is available on Github
Understanding how the board communicates with the app
The first step is to download the APK (search for “net-ecthk-dartsconnect.apk”), and disassemble it. To do this, I used Apktool
apktool d ./net-ecthk-dartsconnect.apk
At first I tried to use JADX to decompile the .smali files back to Java. But I soon realized that the code I was interested in wasn’t there. Looking at the file names, there are many clues that this is a Unity project. And so all the logic of the application isn’t in the Java glue code, but embedded in a DLL.
The heart of the app is located in /assets/bin/Data/Managed/Assembly-CSharp.dll. Using a tool like ILSpy, the DLL can be decompiled into C# code.
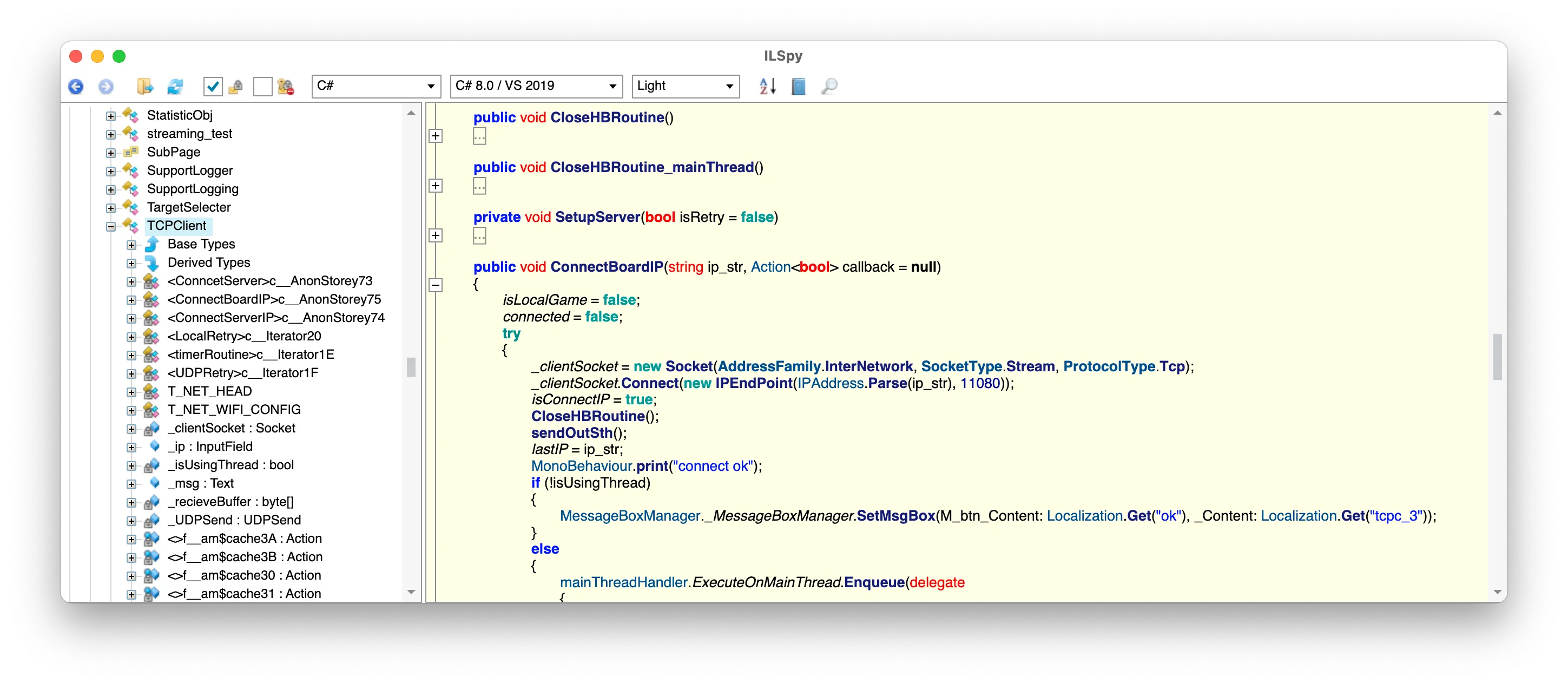
Lets start our investigation. Among all the classes, one looks promising: TCPClient.
From the function ConnectBoardIP
, we learn that the app connects to the board via TCP on port 11080. And that the received data are handled in a function called ReceiveCallback
.
public void ConnectBoardIP(string ip_str, Action<bool> callback = null)
{
//...
try
{
_clientSocket = new Socket(AddressFamily.InterNetwork, SocketType.Stream, ProtocolType.Tcp);
_clientSocket.Connect(new IPEndPoint(IPAddress.Parse(ip_str), 11080));
//...
}
//...
_clientSocket.BeginReceive(_recieveBuffer, 0, _recieveBuffer.Length, SocketFlags.None, ReceiveCallback, null);
}
ReceiveCallback
contains the logic to decode the messages sent by the dartboard. Or at least to extract the correct data based on the message type. For messages of type info and subtype data, four bytes are stored in data_process
and the boolean getkey
is set to true.
private void ReceiveCallback(IAsyncResult AR)
{
//...
byte[] array = new byte[num];
Buffer.BlockCopy(_recieveBuffer, 0, array, 0, num);
input = ByteArrayToString(array);
string[] array2 = input.Split('-');
if (array2[0] != "23" && array2[1] != "08")
{
//...
}
else
{
data_offset = 0;
byte[] value = new byte[4]
{
array[12],
array[13],
array[14],
array[15]
};
int num2 = BitConverter.ToInt32(value, 0);
string text = array2[7] + array2[6] + array2[5] + array2[4];
string text2 = array2[11] + array2[10] + array2[9] + array2[8];
switch (text)
{
//...
case "00000001":
actural_type = "info";
switch (text2)
{
case "00000102":
actural_sub_type = "image";
imageData = new byte[num2 - 20];
Buffer.BlockCopy(array, 40, imageData, 0, num - 40);
data_offset = num - 40;
total_size = num2 + 20 - num;
data_process = new byte[4];
Buffer.BlockCopy(array, 24, data_process, 0, 4);
getkey = true;
break;
case "00000101":
actural_sub_type = "data";
data_process = new byte[4];
Buffer.BlockCopy(array, 20, data_process, 0, 4);
data_offset = num - 20;
total_size = num2 + 20 - num;
getkey = true;
break;
case "00000104":
actural_sub_type = "data";
nextbool = true;
getkey = true;
break;
}
break;
//...
}
//...
}
If we look were these variables are used, we land on the CheckLocalInputChange
method of GamePlayUIManager
. And then on findmapping
and keyMap2
.
private void CheckLocalInputChange()
{
//...
if (_tcpclient.getkey)
{
//...
int keyvalue = BitConverter.ToInt32(_tcpclient.data_process, 0);
int num = findmapping(keyvalue);
string[] array = keyMap2[num].Split(';');
activeGameFlow(array[1], array[2]);
_tcpclient.getkey = false;
}
}
public int findmapping(int keyvalue)
{
string text = string.Empty + keyvalue;
for (int i = 0; i < keyMap2.Length; i++)
{
string text2 = keyMap2[i];
string[] array = text2.Split(';');
if (array[0] == text)
{
return i;
}
}
return -1;
}
public string[] keyMap2 = new string[84]
{
"65;5;3", "66;7;3", "67;3;3", "68;9;3", "69;1;3", "70;11;3", "71;2;3", "72;10;3", "73;4;3", "74;8;3",
"75;6;3", "76;18;3", "77;14;3", "78;13;3", "79;16;3", "80;15;3", "81;19;3", "82;12;3", "83;17;3", "84;20;3",
"89;25;3", "33;5;2", "34;7;2", "35;3;2", "36;9;2", "37;1;2", "38;11;2", "39;2;2", "40;10;2", "41;4;2",
"42;8;2", "43;6;2", "44;18;2", "45;14;2", "46;13;2", "47;16;2", "48;15;2", "49;19;2", "50;12;2", "51;17;2",
"52;20;2", "57;25;2", "129;5;1", "130;7;1", "131;3;1", "132;9;1", "133;1;1", "134;11;1", "135;2;1", "136;10;1",
"137;4;1", "138;8;1", "139;6;1", "140;18;1", "141;14;1", "142;13;1", "143;16;1", "144;15;1", "145;19;1", "146;12;1",
"147;17;1", "148;20;1", "153;25;1", "1;5;1", "2;7;1", "3;3;1", "4;9;1", "5;1;1", "6;11;1", "7;2;1",
"8;10;1", "9;4;1", "10;8;1", "11;6;1", "12;18;1", "13;14;1", "14;13;1", "15;16;1", "16;15;1", "17;19;1",
"18;12;1", "19;17;1", "20;20;1", "25;25;1"
};
keyMap2
is the most interesting part. It allows us to associate each key value with a specific cell on the dartboard. For example “65;5;3” means that key 65 is triple 5, “57;25;2” that key 57 is double bull, etc.
We can connect to the dartboard in two ways. Either directly through the board’s WiFi access point (the factory setting) or through another AP (such as the home WiFi) by setting its SSID and Password in the board’s configuration.
To set the config, we must connect the device to the board AP and send the appropriate message. Then the board will reboot and try to connect to the newly set AP.
The class TCPClient
contains a method setWifi
and two inner classes T_NET_HEAD
and T_NET_WIFI_CONFIG
. That’s all we need to understand how to build the WiFi configuration message.
public void setWifi(string id, string pw)
{
T_NET_WIFI_CONFIG t_NET_WIFI_CONFIG = new T_NET_WIFI_CONFIG(id, pw);
SendData(t_NET_WIFI_CONFIG.tobyteArray());
}
private class T_NET_HEAD
{
private int magic_code = 2083;
private int type;
private int sub_type;
private int size;
private int reserved;
public T_NET_HEAD(int type, int sub_type, int size, int reserved)
{
this.type = type;
this.sub_type = sub_type;
this.size = size;
this.reserved = reserved;
}
public byte[] tobyteArray()
{
byte[] array = new byte[20];
byte[] bytes = BitConverter.GetBytes(magic_code);
Buffer.BlockCopy(bytes, 0, array, 0, bytes.Length);
byte[] bytes2 = BitConverter.GetBytes(type);
Buffer.BlockCopy(bytes2, 0, array, 4, bytes2.Length);
byte[] bytes3 = BitConverter.GetBytes(sub_type);
Buffer.BlockCopy(bytes3, 0, array, 8, bytes3.Length);
byte[] bytes4 = BitConverter.GetBytes(size);
Buffer.BlockCopy(bytes4, 0, array, 12, bytes4.Length);
byte[] bytes5 = BitConverter.GetBytes(reserved);
Buffer.BlockCopy(bytes4, 0, array, 16, bytes5.Length);
return array;
}
}
private class T_NET_WIFI_CONFIG
{
private int reserved;
private int result;
private byte[] ssid;
private byte[] wpapsk;
private T_NET_HEAD head;
public T_NET_WIFI_CONFIG(string ssid_string, string wpapsk_string)
{
reserved = 0;
result = 0;
ssid = new byte[64];
wpapsk = new byte[64];
byte[] bytes = Encoding.Default.GetBytes(ssid_string);
Buffer.BlockCopy(bytes, 0, ssid, 0, bytes.Length);
byte[] bytes2 = Encoding.Default.GetBytes(wpapsk_string);
Buffer.BlockCopy(bytes2, 0, wpapsk, 0, bytes2.Length);
head = new T_NET_HEAD(2, 259, 136, 0);
}
public byte[] tobyteArray()
{
byte[] array = new byte[156];
Buffer.BlockCopy(head.tobyteArray(), 0, array, 0, 20);
byte[] bytes = BitConverter.GetBytes(reserved);
Buffer.BlockCopy(bytes, 0, array, 20, bytes.Length);
byte[] bytes2 = BitConverter.GetBytes(result);
Buffer.BlockCopy(bytes2, 0, array, 24, bytes2.Length);
Buffer.BlockCopy(ssid, 0, array, 28, ssid.Length);
Buffer.BlockCopy(wpapsk, 0, array, 92, wpapsk.Length);
MonoBehaviour.print("sent out ssid:" + ByteArrayToString(ssid));
MonoBehaviour.print("sent out wpapsk:" + ByteArrayToString(wpapsk));
string text = ByteArrayToString(array);
MonoBehaviour.print("sent out hearder:" + text);
return array;
}
}
Another interesting method is keepSendingHeartBeat. By sending a heartbeat message and waiting for an ack from the board, we can monitor the connection and detect if a disconnection occurs.
public void (object State)
{
if (connected)
{
T_NET_HEAD t_NET_HEAD = new T_NET_HEAD(255, 0, 0, 0);
SendData(t_NET_HEAD.tobyteArray());
}
else if (isConnectIP)
{
T_NET_HEAD t_NET_HEAD2 = new T_NET_HEAD(255, 0, 0, 0);
SendData(t_NET_HEAD2.tobyteArray());
}
}
Developing a mobile app
With all the information gathered earlier (TCP on port 11080, the messages types and binary structure, the mapping of the dartboard wedges/cells) it’s not too difficult to rebuild the equivalent in Kotlin. Thanks to Kotlin and Compose Multiplatform, the app runs on both Android and iOS.
The source code is available on Github
Currently, the app is very basic and has many limitations but it’s a good proof of concept and it offer a good starting point to build a more complete version. I’ll probably add a few features every time friends come over for a night of darts.
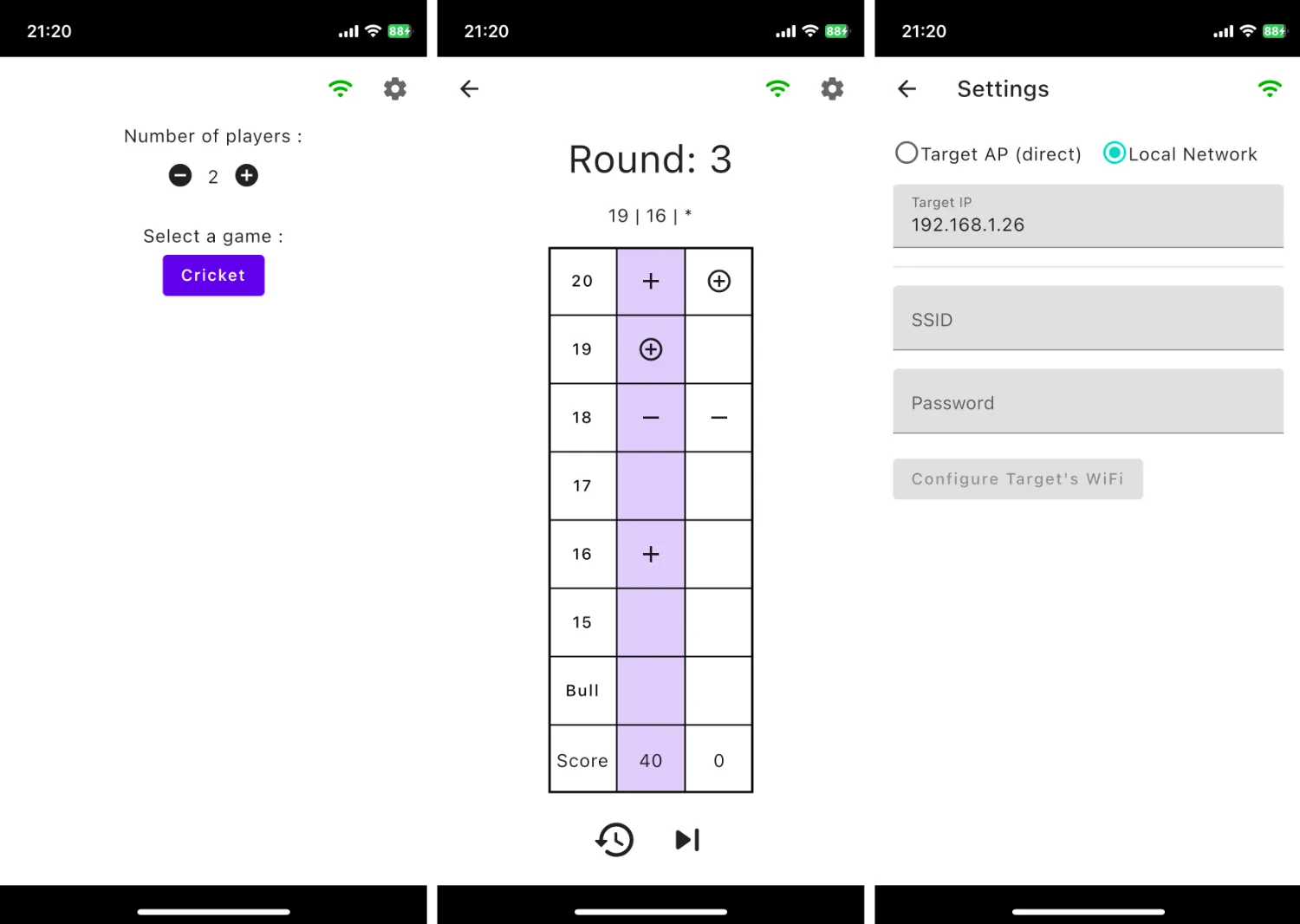
Wrap up
I’ve done way more development for iOS than for Android. And a few years ago I hated developing apps for Android. Java, the XML layout system and the bindings, the lifecycle of activities and fragments, the way screen rotation is handled, the audio background services, and an infinity of others stuffs, all of it made me feel dirty. Everything seemed too verbose and over-enginered.
Things were far from perfect on iOS either, but SwiftUI, was a breath of fresh air despite all its early flaws. One thing I hate, though, is how tightly tied the SwiftUI code is to the iOS version. Way to often, you can’t use a useful modifier because it’s not available on the lowest iOS version you’re targeting. And even if it’s just a slight change in the function signature you’re stuck with the old syntax or have to clutter your code with #available(...)
statments. And since you can’t use them in the middle of a modifier chain, you end up duplicating a lot of code.
Over the past year I’ve worked on several projects using JetPack Compose, and I have to say that I really enjoyed it. I can’t really explain why, but I found it more natural and pleasant to work with than SwiftUI. It’s very similar in concept, but the little differences are more in line with how my brain is wired. And overall, it feels like the whole ecosystem is moving in the right direction. Gradle with its many syntax versions and ways to manage dependency versioning, is still far from feeling simple and lightweight. The need to add a new import almost every time you type a word, and end-up with an import section as long as than the actual code is also a pain.
After this little trial with KMP and CMP, I will for sure consider this stack for bigger projects in the future (even if only iOS is targeted).